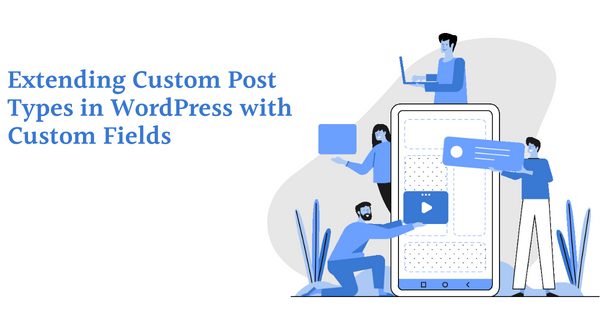
Custom post types in WordPress provide a powerful way to extend the default post and page functionalities, allowing you to create and manage different types of content tailored to your specific needs. However, sometimes you may want to add additional data or fields to your custom post types to enhance their functionality. This is where custom fields come into play. In this article, we will explore how to add custom fields to custom post types in WordPress, along with some practical examples.
Understanding Custom Fields
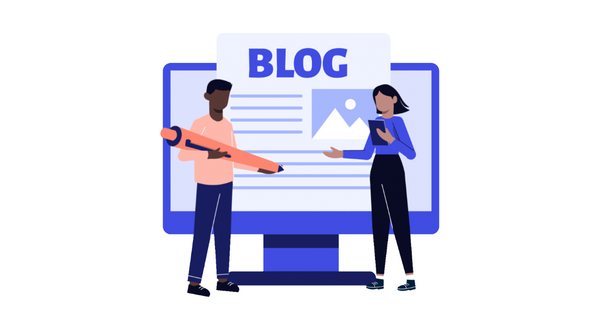
Custom fields, also known as post meta, are additional data points that can be associated with posts, pages, or custom post types in WordPress. They allow you to store and retrieve extra information that is not part of the standard content fields like the title or the body. Custom fields are stored in the database and can be accessed programmatically to display or manipulate the data associated with a post.
Defining Custom Post Types
Before diving into adding custom fields, let’s quickly recap how to define custom post types in WordPress. You can register custom post types using the register_post_type()
function. Within the registration process, you specify various parameters such as the name, labels, supported features, and more. Once you have registered a custom post type, it becomes available in the WordPress admin area, enabling you to create and manage the content of that type.
Adding Custom Fields to Custom Post Types
To add custom fields to your custom post types, you can use the register_meta()
function in WordPress. This function allows you to define and register custom fields, specifying their attributes such as the type, description, and whether they should be displayed in the Gutenberg editor.
Let’s consider an example where we have two custom post types: “Books” and “Movies”. Each post type requires different sets of custom fields. For the “Books” post type, we want to add a dropdown field for the genre and a single-line text area for the author’s name. For the “Movies” post type, we need a dropdown field for the genre, a text field for the director, and a text field for the release year.
In your theme’s functions.php
file, you can add the following code to register the custom fields:
// Add custom fields to the "Books" post type
function register_custom_fields_for_books() {
$args = array(
'type' => 'string',
'description' => 'Genre',
'single' => true,
'show_in_rest' => true,
);
register_meta('post', 'book_genre', $args);
$args = array(
'type' => 'string',
'description' => "Author's Name",
'single' => true,
'show_in_rest' => true,
);
register_meta('post', 'author_name', $args);
}
add_action('init', 'register_custom_fields_for_books');
// Add custom fields to the "Movies" post type
function register_custom_fields_for_movies() {
$args = array(
'type' => 'string',
'description' => 'Genre',
'single' => true,
'show_in_rest' => true,
);
register_meta('post', 'movie_genre', $args);
$args = array(
'type' => 'string',
'description' => 'Director',
'single' => true,
'show_in_rest' => true,
);
register_meta('post', 'director_name', $args);
$args = array(
'type' => 'string',
'description' => 'Release Year',
'single' => true,
'show_in_rest' => true,
);
register_meta('post', 'release_year', $args);
}
add_action('init', 'register_custom_fields_for_movies');
In this code snippet, we define two functions: register_custom_fields_for_books()
and register_custom_fields_for_movies()
. Each function registers the respective custom fields for the corresponding post type using the register_meta()
function.
Summary
Adding custom fields to your custom post types can greatly enhance their functionality and enable you to store and display additional data specific to your content. By utilizing the register_meta()
function in WordPress, you can easily define and register custom fields, tailoring them to meet the requirements of each post type. Whether it’s a dropdown, a text area, or any other field type, custom fields provide a flexible way to extend your content management capabilities in WordPress. So go ahead and unleash the power of custom fields to unlock new possibilities for your custom post types.
Comments (1)